In this IoT project, I have explained how to make an Arduino Cloud ESP8266 home automation system to control appliances through the internet from anywhere in the world.
With this Internet of Things project, you can control 4 relays from the Arduino IoT Cloud dashboard, Google Home/Alexa app, and manual pushbuttons.
If there is no internet available, you can still control the appliances with push buttons.
I have used all the FREE tools for this voice control smart home IoT project.
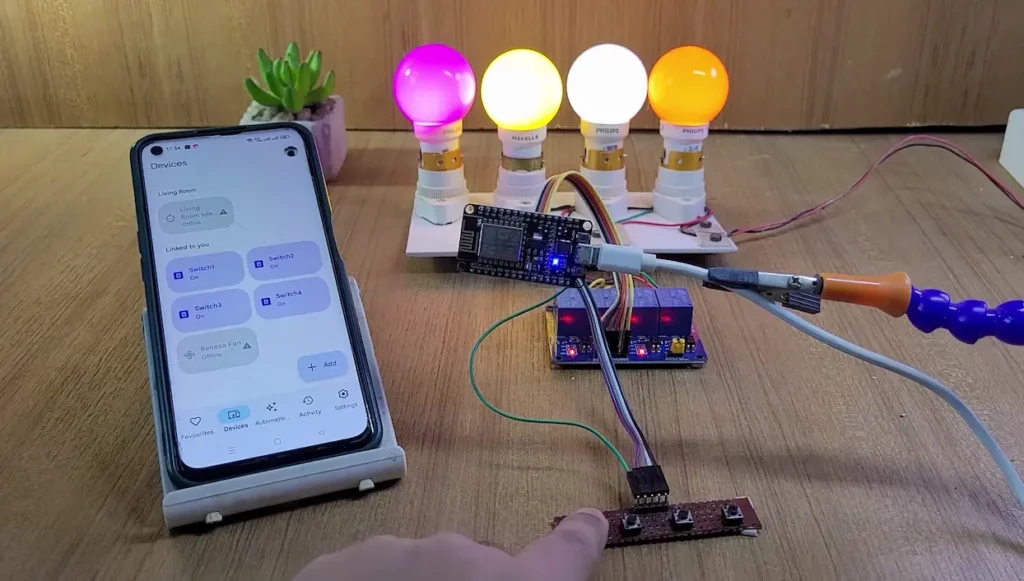
So if you follow all the steps, you can easily make this Home Automation System with Arduino IoT Cloud and ESP32 for your home.
Table of Contents
Required Components for the ESP8266 IoT projects
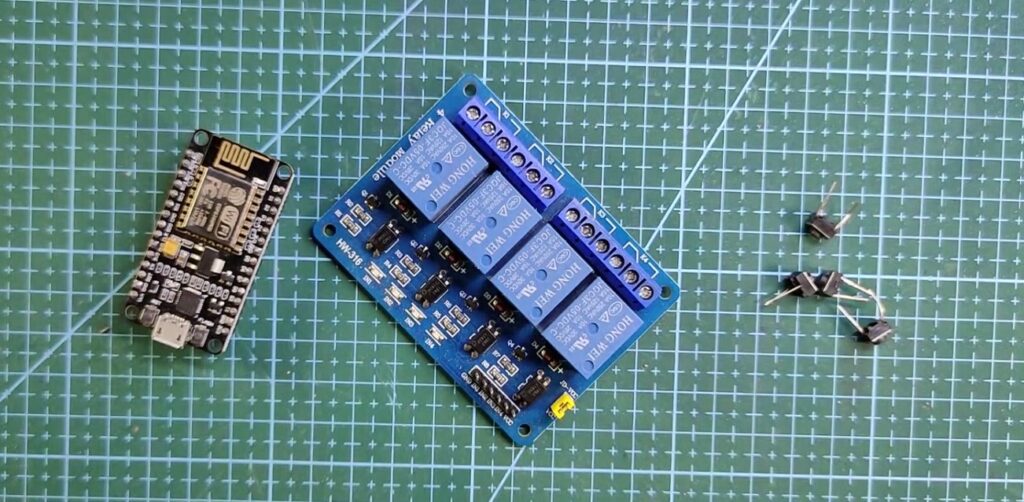
- ESP8266 NodeMCU
- 4-channel 5V SPDT Relay Module (Active LOW)
- Push buttons
Circuit of the Arduino Cloud NodeMCU project
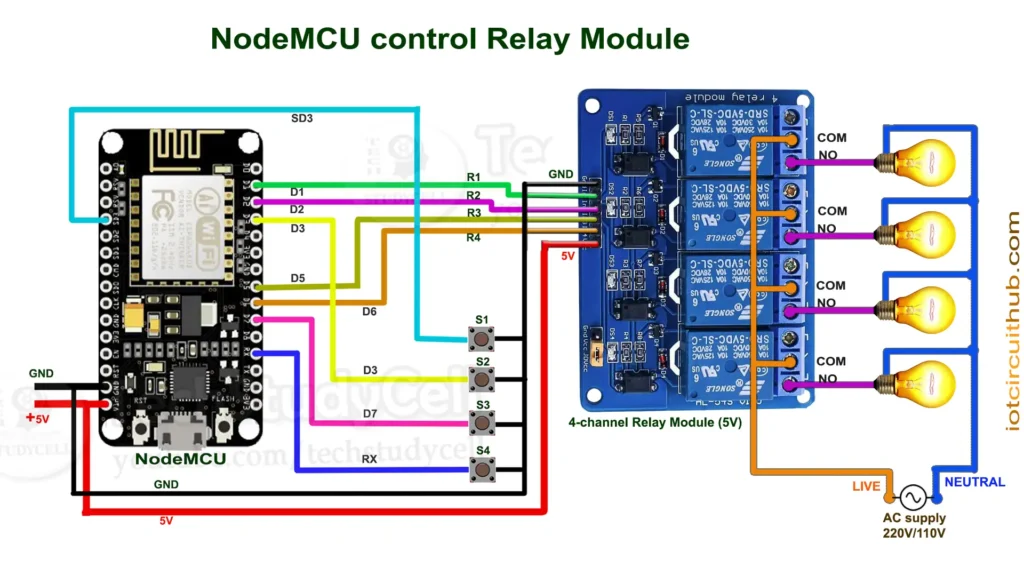
In the circuit, I have used D1 (GPIO-5), D2 (GPIO-4), D5 (GPIO-14), and D6 (GPIO-12) GPIO pins to control the 4-channel relay module.
And the GPIO SD3 (GPIO-10), D3 (GPIO-0), D7 (GPIO-13), and RX (GPIO-3) connected with the pushbuttons to control the relay module manually.
I have used the INPUT_PULLUP function in Arduino IDE instead of using the pull-up resistors with each button.
As per the source code, when the control pins of the relay module receive a LOW signal the relay will turn on and the relay will turn off for the HIGH signal in the control pin.
I have used a 5V 5Amp mobile charger to supply the circuit.
Please take proper safety precautions while connecting the AC appliances.
Tutorial Video on ESP8266 Arduino IoT Cloud
In the Arduino Cloud tutorial, I have covered the following steps in detail.
- Create new things in Arduino IoT Cloud.
- How to set up Arduino IoT Cloud Dashboard.
- How to set up Arduino IoT Cloud for ESP8266.
- Programming the ESP8266 NodeMCU with Arduino IDE
- Connect Arduino IoT Cloud with Google Home App.
Arduino IoT Cloud FREE account setup
For this IoT project, I have used the Arduino Cloud Free plan.
First, you have to add an ESP8266 device to Arduino IoT Cloud.
Then you must add 4 Google/Alexa-compatible switch variables to the Arduino Cloud Thing.
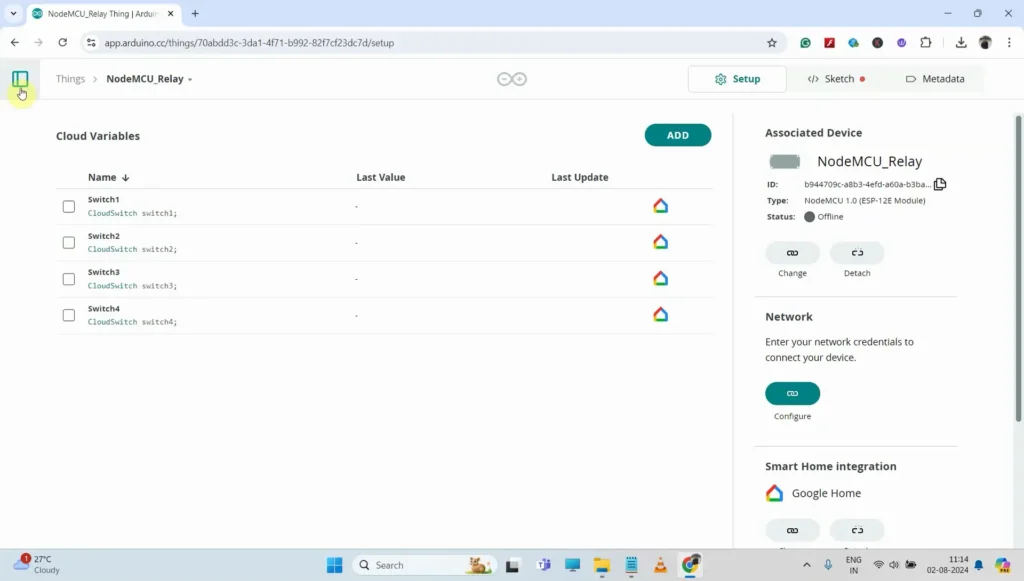
Please click on the following link for more details on Arduino IoT Cloud setup.
Getting Started with Arduino IoT Cloud
Program NodeMCU with Arduino IDE
In the tutorial video, I have explained all the steps to program the NodeMCU using Arduino IDE.
- Update the Preferences –> Aditional boards Manager URLs: https://dl.espressif.com/dl/package_esp32_index.json, http://arduino.esp8266.com/stable/package_esp8266com_index.json
- Then install the ESP8266 board from the Board manager or Click Here to download the ESP8266 board.
- Install all the required libraries in Arduino IDE:
- ArduinoIoTCloud by Arduino and all the dependencies.
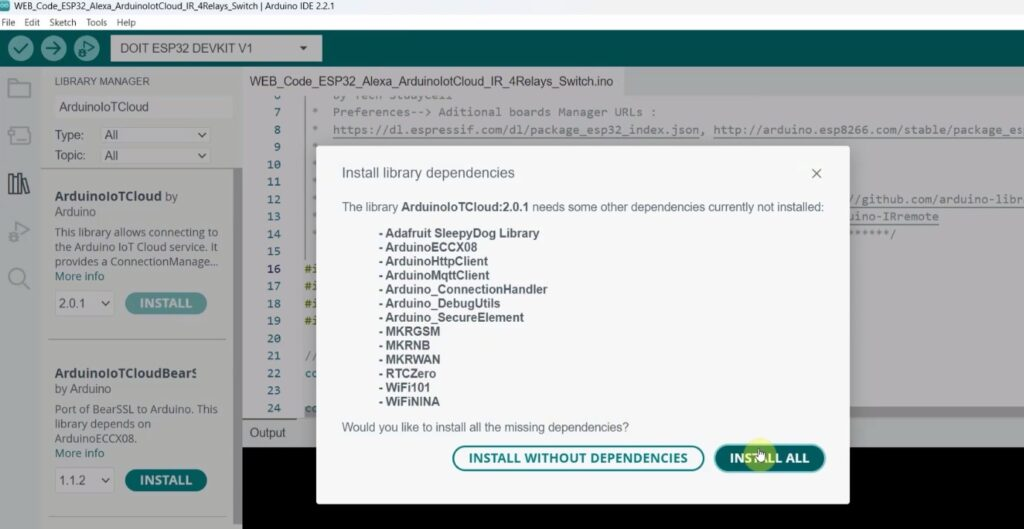
When you try to install the ArduinoIoTCloud library, it will ask you to install all the dependencies. Then Click on Install All.
Source Code for Arduino IoT Cloud ESP8266 home automation
#include <ArduinoIoTCloud.h>
#include <Arduino_ConnectionHandler.h>
#include <ESP8266Wifi.h>
const char DEVICE_LOGIN_NAME[] = ""; //Enter DEVICE ID
const char SSID[] = ""; //Enter WiFi SSID (name)
const char PASS[] = ""; //Enter WiFi password
const char DEVICE_KEY[] = ""; //Enter Secret device password (Secret Key)
// define the GPIO connected with Relays and switches
#define RelayPin1 5 //D1
#define RelayPin2 4 //D2
#define RelayPin3 14 //D5
#define RelayPin4 12 //D6
#define SwitchPin1 10 //SD3
#define SwitchPin2 0 //D3
#define SwitchPin3 13 //D7
#define SwitchPin4 3 //RX
#define wifiLed 16 //D0
int toggleState_1 = 0; //Define integer to remember the toggle state for relay 1
int toggleState_2 = 0; //Define integer to remember the toggle state for relay 2
int toggleState_3 = 0; //Define integer to remember the toggle state for relay 3
int toggleState_4 = 0; //Define integer to remember the toggle state for relay 4
void onSwitch1Change();
void onSwitch2Change();
void onSwitch3Change();
void onSwitch4Change();
CloudSwitch switch1;
CloudSwitch switch2;
CloudSwitch switch3;
CloudSwitch switch4;
void initProperties(){
ArduinoCloud.setBoardId(DEVICE_LOGIN_NAME);
ArduinoCloud.setSecretDeviceKey(DEVICE_KEY);
ArduinoCloud.addProperty(switch1, READWRITE, ON_CHANGE, onSwitch1Change);
ArduinoCloud.addProperty(switch2, READWRITE, ON_CHANGE, onSwitch2Change);
ArduinoCloud.addProperty(switch3, READWRITE, ON_CHANGE, onSwitch3Change);
ArduinoCloud.addProperty(switch4, READWRITE, ON_CHANGE, onSwitch4Change);
}
WiFiConnectionHandler ArduinoIoTPreferredConnection(SSID, PASS);
const int debounceDelay = 200; // Debounce delay in milliseconds
unsigned long lastDebounceTime1 = 0;
unsigned long lastDebounceTime2 = 0;
unsigned long lastDebounceTime3 = 0;
unsigned long lastDebounceTime4 = 0;
// Track the previous state of each switch to handle edge detection
bool previousSwitchState1 = HIGH;
bool previousSwitchState2 = HIGH;
bool previousSwitchState3 = HIGH;
bool previousSwitchState4 = HIGH;
void manual_control() {
unsigned long currentMillis = millis();
// Manual Switch Control
// Check each switch pin and toggle the corresponding relay if the switch is pressed (LOW state)
// Check if Switch 1 is pressed
if (digitalRead(SwitchPin1) == LOW && previousSwitchState1 == HIGH) {
if (currentMillis - lastDebounceTime1 >= debounceDelay) {
toggleState_1 = !toggleState_1;
digitalWrite(RelayPin1, !toggleState_1);
switch1 = toggleState_1; // Toggle Relay 1
lastDebounceTime1 = currentMillis; // Update the last debounce time
}
}
previousSwitchState1 = digitalRead(SwitchPin1);
// Check if Switch 2 is pressed
if (digitalRead(SwitchPin2) == LOW && previousSwitchState2 == HIGH) {
if (currentMillis - lastDebounceTime2 >= debounceDelay) {
toggleState_2 = !toggleState_2;
digitalWrite(RelayPin2, !toggleState_2);
switch2 = toggleState_2; // Toggle Relay 2
lastDebounceTime2 = currentMillis; // Update the last debounce time
}
}
previousSwitchState2 = digitalRead(SwitchPin2);
// Check if Switch 3 is pressed
if (digitalRead(SwitchPin3) == LOW && previousSwitchState3 == HIGH) {
if (currentMillis - lastDebounceTime3 >= debounceDelay) {
toggleState_3 = !toggleState_3;
digitalWrite(RelayPin3, !toggleState_3);
switch3 = toggleState_3; // Toggle Relay 3
lastDebounceTime3 = currentMillis; // Update the last debounce time
}
}
previousSwitchState3 = digitalRead(SwitchPin3);
// Check if Switch 4 is pressed
if (digitalRead(SwitchPin4) == LOW && previousSwitchState4 == HIGH) {
if (currentMillis - lastDebounceTime4 >= debounceDelay) {
toggleState_4 = !toggleState_4;
digitalWrite(RelayPin4, !toggleState_4);
switch4 = toggleState_4; // Toggle Relay 4
lastDebounceTime4 = currentMillis; // Update the last debounce time
}
}
previousSwitchState4 = digitalRead(SwitchPin4);
}
void setup() {
// Initialize serial and wait for port to open:
Serial.begin(9600);
// This delay gives the chance to wait for a Serial Monitor without blocking if none is found
delay(1500);
// Defined in thingProperties.h
initProperties();
// Connect to Arduino IoT Cloud
ArduinoCloud.begin(ArduinoIoTPreferredConnection);
setDebugMessageLevel(2);
ArduinoCloud.printDebugInfo();
pinMode(RelayPin1, OUTPUT);
pinMode(RelayPin2, OUTPUT);
pinMode(RelayPin3, OUTPUT);
pinMode(RelayPin4, OUTPUT);
pinMode(wifiLed, OUTPUT);
pinMode(SwitchPin1, INPUT_PULLUP);
pinMode(SwitchPin2, INPUT_PULLUP);
pinMode(SwitchPin3, INPUT_PULLUP);
pinMode(SwitchPin4, INPUT_PULLUP);
//During Starting all Relays should TURN OFF
digitalWrite(RelayPin1, HIGH);
digitalWrite(RelayPin2, HIGH);
digitalWrite(RelayPin3, HIGH);
digitalWrite(RelayPin4, HIGH);
digitalWrite(wifiLed, HIGH); //Turn OFF WiFi LED
}
void loop() {
ArduinoCloud.update();
manual_control(); //Control relays manually
if (WiFi.status() != WL_CONNECTED)
{
digitalWrite(wifiLed, HIGH); //Turn OFF WiFi LED
//Serial.println("NO WIFI");
}
else{
digitalWrite(wifiLed, LOW); //Turn ON WiFi LED
//Serial.println("YES WIFI");
}
}
void onSwitch1Change() {
if (switch1 == 1)
{
digitalWrite(RelayPin1, LOW);
Serial.println("Device1 ON");
toggleState_1 = 1;
}
else
{
digitalWrite(RelayPin1, HIGH);
Serial.println("Device1 OFF");
toggleState_1 = 0;
}
}
void onSwitch2Change() {
if (switch2 == 1)
{
digitalWrite(RelayPin2, LOW);
Serial.println("Device2 ON");
toggleState_2 = 1;
}
else
{
digitalWrite(RelayPin2, HIGH);
Serial.println("Device2 OFF");
toggleState_2 = 0;
}
}
void onSwitch3Change() {
if (switch3 == 1)
{
digitalWrite(RelayPin3, LOW);
Serial.println("Device2 ON");
toggleState_3 = 1;
}
else
{
digitalWrite(RelayPin3, HIGH);
Serial.println("Device3 OFF");
toggleState_3 = 0;
}
}
void onSwitch4Change() {
if (switch4 == 1)
{
digitalWrite(RelayPin4, LOW);
Serial.println("Device4 ON");
toggleState_4 = 1;
}
else
{
digitalWrite(RelayPin4, HIGH);
Serial.println("Device4 OFF");
toggleState_4 = 0;
}
}
After uploading the code to NodeMCU, please refer to the following articles for connecting the Arduino IoT Cloud Account with Google Home or Amazon Alexa App.
After doing all these steps, now you control the appliances either with Google Assistant or Alexa as per the configuration in Arduino IoT Cloud.
PCB for the ESP8266 IoT Projects
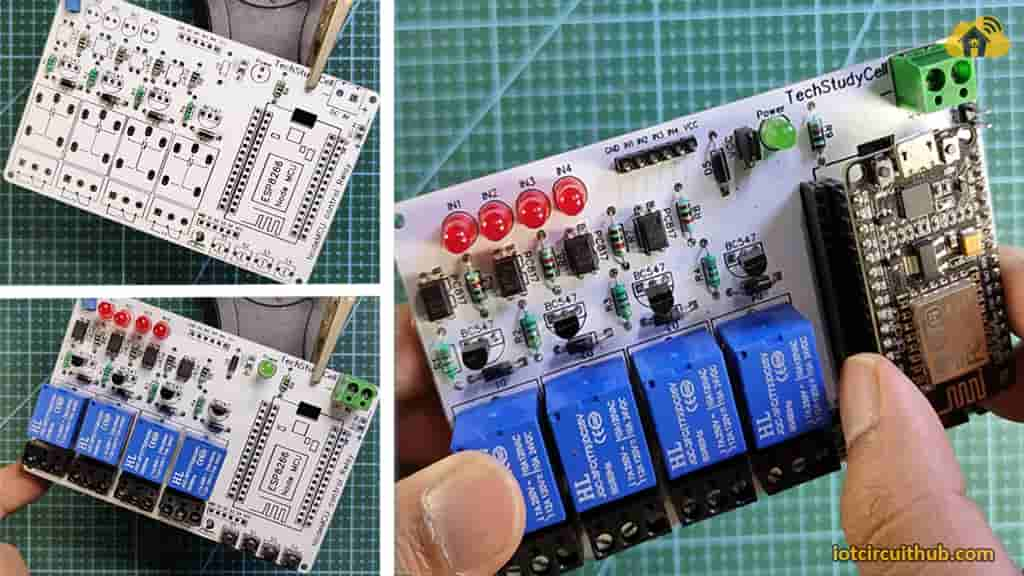
You can also use this PCB for any NodeMCU IoT project. Just download the Gerber file and order the PCB from PCBWay.
ou can order any custom-designed PCBs from PCBWay at very reasonable prices.

PCBWay not only produces FR-4 and Aluminum boards but also advanced PCBs like Rogers, HDI, and Flexible and Rigid-Flex boards, at very affordable prices.
For the online instant quote page please visit – pcbway.com/orderonline
You can also explore different PCB projects from their Open-source community pcbway.com/project/.
For more details please visit the following articles.
Why PCBway
Control Relay with Google Assistant or Alexa app
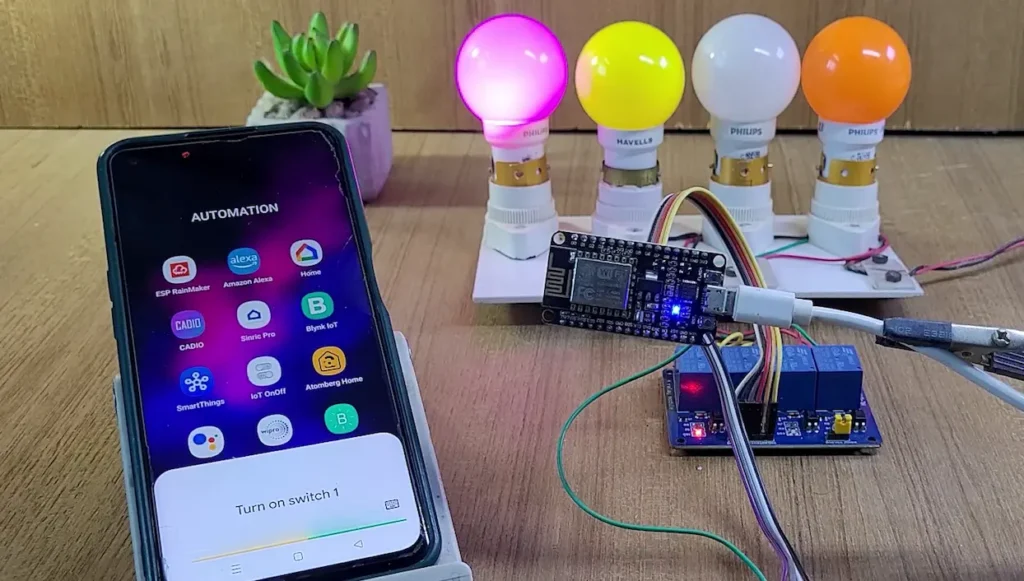
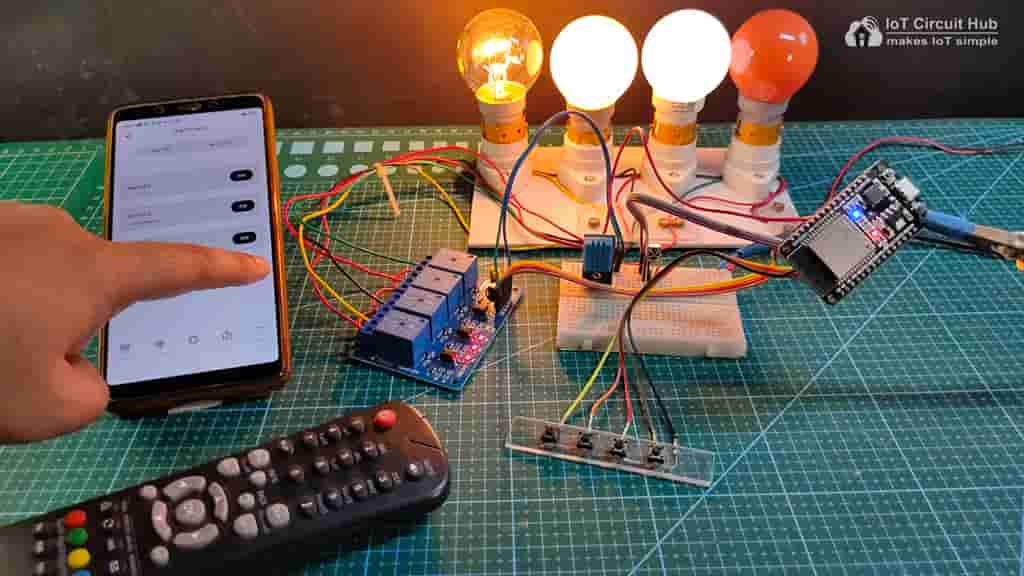
If the ESP32 is connected to the WiFi, then you can ask Google Assistant/Alexa, to turn on the switch [“Alexa, Turn ON Switch-1”]. Thus, you can control appliances like lights, fans, etc with voice commands using the Google Home/Amazon Alexa App from anywhere in the world.
You can also monitor the real-time feedback and room temperature in the Google Home or Amazon Alexa app.
Control Relays with Arduino IoT Cloud Remote App
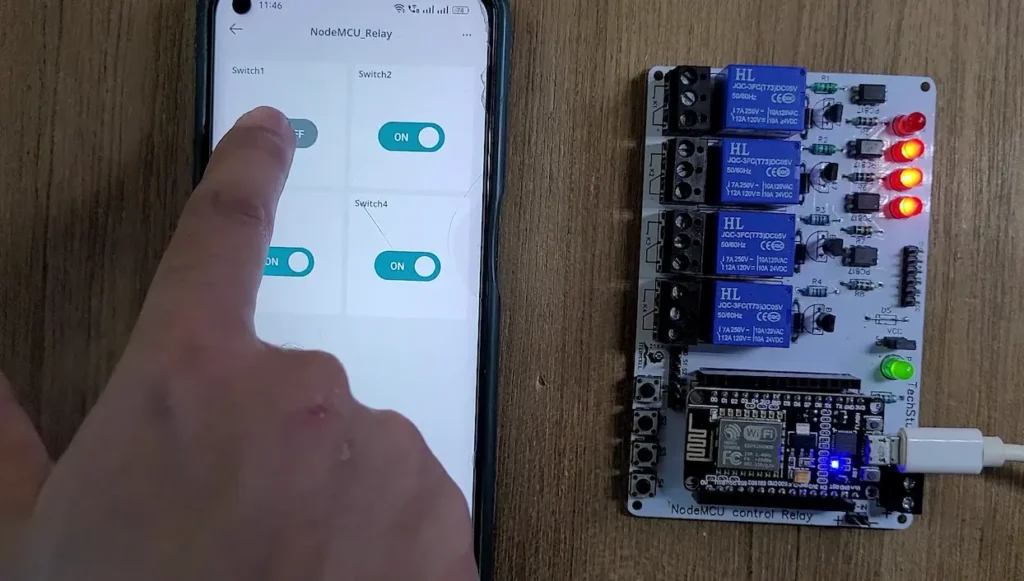
You can also control the relays and monitor real-time feedback from the Arduino IoT Cloud Remote App.
Just download and install the Arduino IoT Cloud Remote app from Google Play Store or App Store, then log in to your Arduino Cloud account and select the dashboard.
Control Relays with Manual Buttons
You can always control the appliances manually with buttons. if the NodeMCU is not connected to the Wi-Fi, still you can control the appliances with buttons.
I hope you like this Smart house IoT project idea with the Arduino Cloud NodeMCU ESP8266.
Click Here for more such ESP8266 projects.
Please do share your feedback on this IoT project. Thank you for your time.